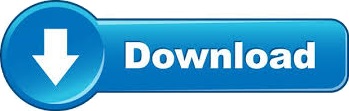

If you want to improve on this you could use a heuristic to improve on the result, for example:
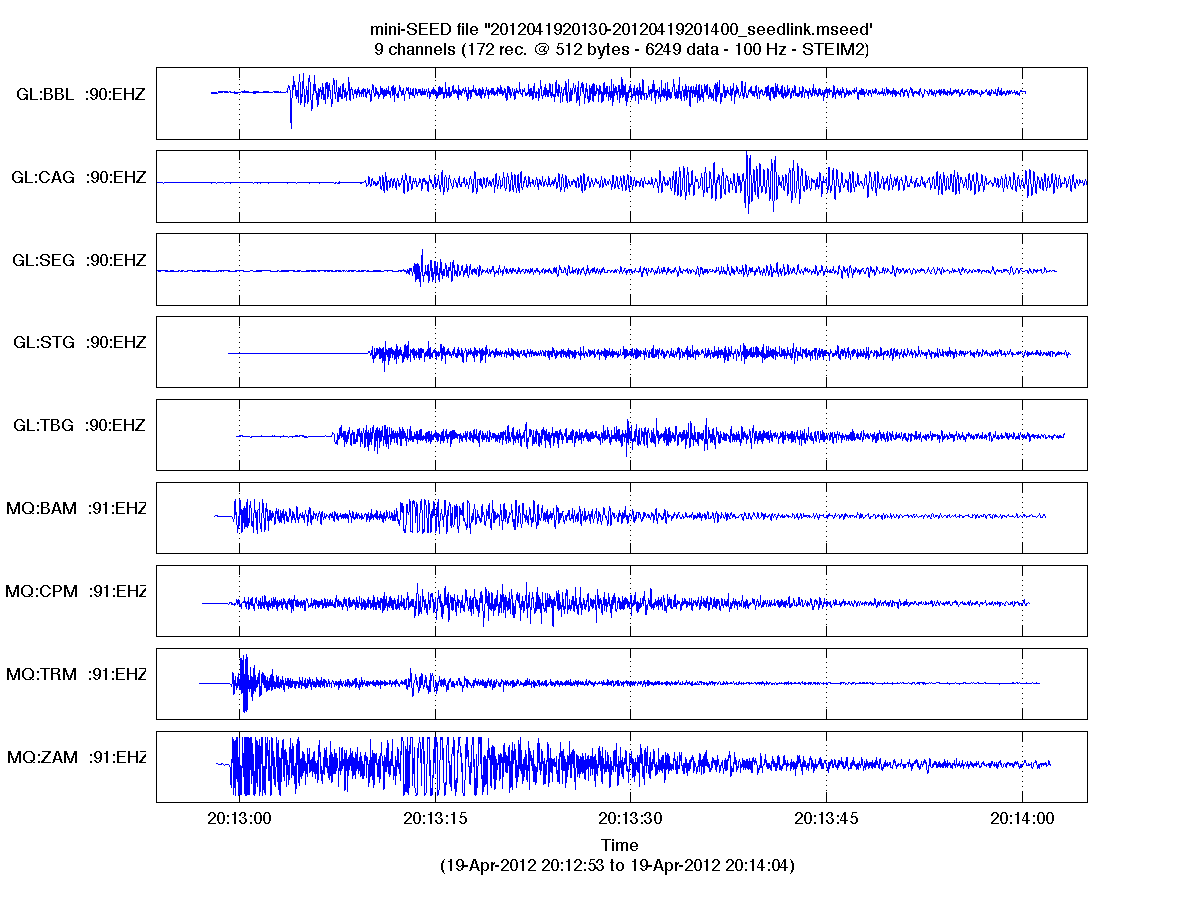
It will not be optimal but probably not very bad. Keep repeating the previous step untill there are k points in the set.Add the furthest point to the set (increase the distance most from current points in set).Add 1 point to the empty set, preferably one in the corner or in the edge (Of course you can just try all of them).outputImage = inputImage(rowIndex,colIndex,:) įinally, the new upsampled image is created by simply indexing into the original image.Assuming you are just looking for a decent solution I would recommend a simple solution similar to the "furthest insertion" starting position for the travelling salesman problem: The call to min ensures that none of these indices are larger than the original image size oldSize(.). These coordinates are then divided by the scale factor to give a set of pixel-center coordinates for the original image, which then have 0.5 added to them and are rounded off to get a set of integer indices for the original image. The “coordinate” of the pixel is thus treated as the center, which is why 0.5 is subtracted from the indices. Each image pixel is considered as having a given width, such that pixel 1 spans from 0 to 1, pixel 2 spans from 1 to 2, etc. First, a set of indices for the upsampled image is computed: 1:newSize(.). Next, a new set of indices is computed for both the rows and columns. If the scale factor is less than 1 you could end up with a weird case of one of the size values being 0, which is why the call to max is there to replace anything less than 1 with 1. This result is rounded down to the nearest integer with floor. In case anyone is interested, I thought I’d explain how the solution above works… newSize = max(floor(scale.*oldSize(1:2)),1) įirst, to get the new row and column sizes the old row and column sizes are multiplied by the scale factor. OutputImage = inputImage(rowIndex,colIndex,:) Īnother option would be to use the built-in interp2 function, although you mentioned not wanting to use built-in functions in one of your comments. NewSize = max(floor(scale.*oldSize(1:2)),1) %# Compute the new image size OldSize = size(inputImage) %# Get the size of your image Scale = %# The resolution scale factors: Here’s how it would be applied to your problem: %# Initializations:
NEAREST NEIGHBOR MATLAB 2012 CODE
Jj = round( (j-1)*(c-1)/(scale(2)*c-1)+1 ) Ī while back I went through the code of the imresize function in the MATLAB Image Processing Toolbox to create a simplified version for just nearest neighbor interpolation of images. % map from output image location to input image location OutputI = zeros(scale(1)*r,scale(2)*c, class(inputI))
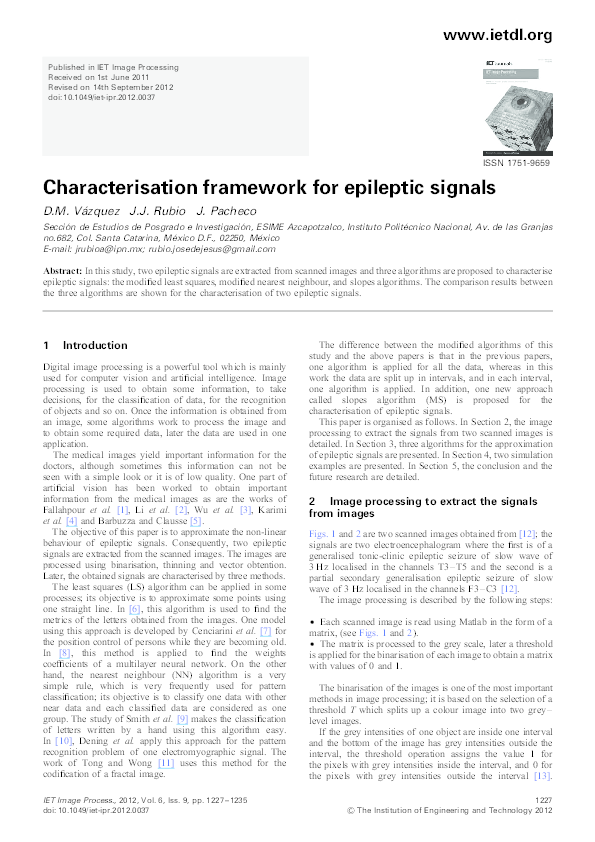
Scale = % you could scale each dimension differently Putting pieces together, we get the following code: % read a sample image Therefore substituting for each gives us: jj = (j-1)*(c-1)/(scaleC*c-1) + 1 In our case, x is the i/ j coordinate and y is the ii/ jj coordinate. Since this is a simple mapping we use the formula that maps a given x to y (given all the other params): x-minX y-minY The idea is that for each location (i,j) in the output image, we want to map it to the “nearest” location in the input image coordinates. Note: I am using matrix notation (row/col), so: To illustrate, consider the following notation: 1 c 1 scaleC*c What you should be doing is the opposite (from output to input). Consider an example where input image is all white and output initialized to black, we get the following: Now the problem with your code is that you are mapping locations from the input image to the output image, which is why you are getting the spotty output. In case you are trying to understand how it works, keep reading… I think gnovice‘s solution is best in that regard. This answer is more explanatory than trying to be concise and efficient.
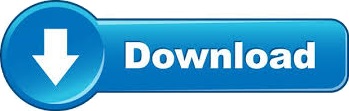